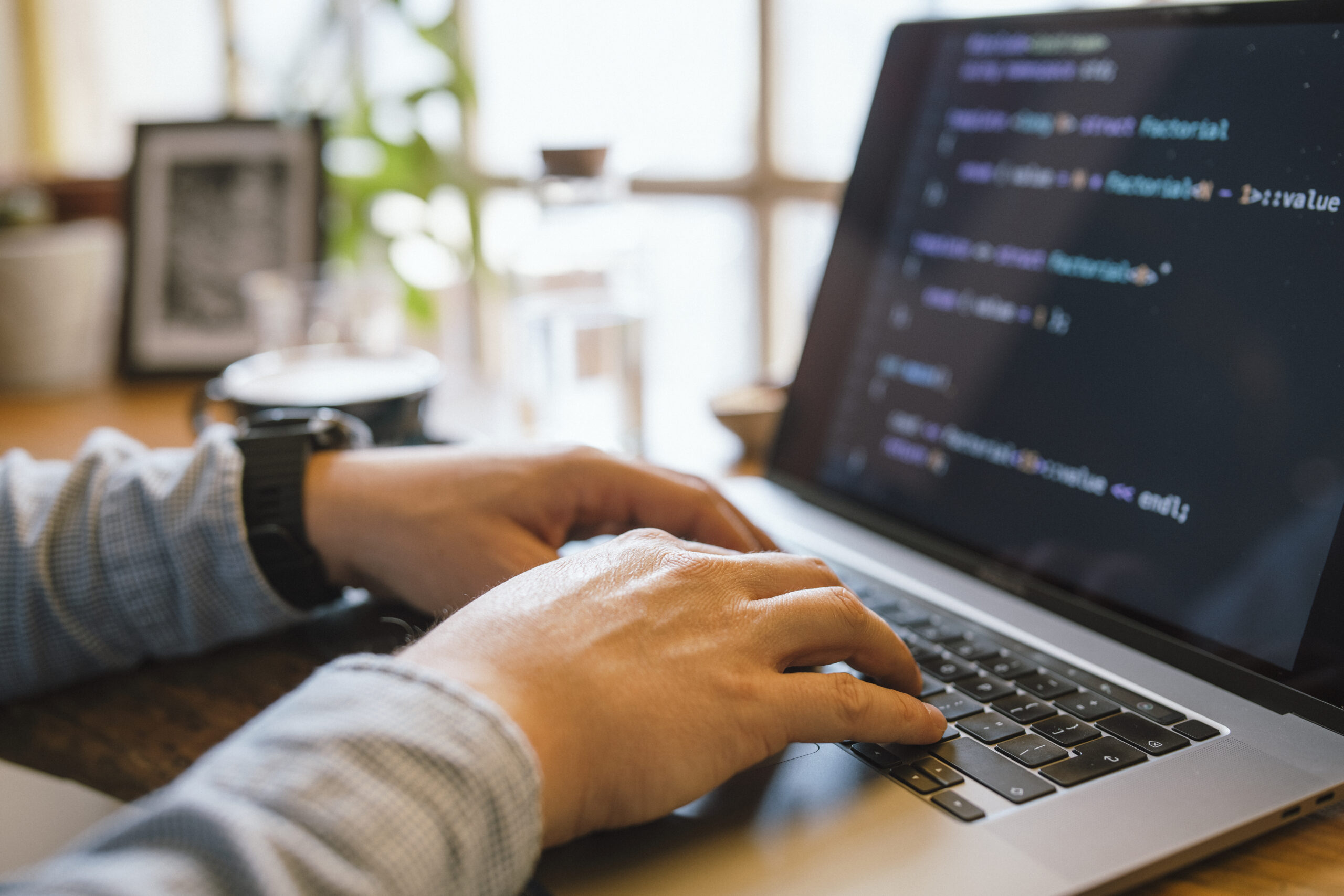
Debugging is One of the more vital — nonetheless frequently disregarded — techniques in a very developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about understanding how and why issues go Improper, and Understanding to Feel methodically to resolve difficulties proficiently. No matter whether you are a novice or possibly a seasoned developer, sharpening your debugging capabilities can preserve hrs of disappointment and drastically boost your productivity. Listed here are several strategies to help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest approaches developers can elevate their debugging abilities is by mastering the applications they use on a daily basis. When composing code is a single part of enhancement, being aware of the best way to interact with it correctly for the duration of execution is equally vital. Modern-day advancement environments come Outfitted with potent debugging abilities — but a lot of developers only scratch the floor of what these resources can perform.
Get, for instance, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools enable you to set breakpoints, inspect the worth of variables at runtime, step by way of code line by line, as well as modify code to the fly. When made use of properly, they Permit you to observe accurately how your code behaves for the duration of execution, that is a must have for tracking down elusive bugs.
Browser developer instruments, including Chrome DevTools, are indispensable for entrance-conclude builders. They help you inspect the DOM, observe network requests, see serious-time effectiveness metrics, and debug JavaScript inside the browser. Mastering the console, sources, and community tabs can turn discouraging UI problems into workable responsibilities.
For backend or program-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Command in excess of operating procedures and memory administration. Studying these equipment can have a steeper Studying curve but pays off when debugging performance troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, become relaxed with Model Command methods like Git to comprehend code heritage, obtain the exact moment bugs had been introduced, and isolate problematic adjustments.
Eventually, mastering your instruments suggests likely further than default configurations and shortcuts — it’s about developing an intimate knowledge of your improvement surroundings in order that when troubles come up, you’re not dropped at nighttime. The higher you recognize your equipment, the more time you'll be able to devote solving the actual problem rather than fumbling through the procedure.
Reproduce the condition
One of the more important — and sometimes neglected — measures in efficient debugging is reproducing the issue. Before leaping in the code or producing guesses, developers have to have to produce a regular surroundings or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug will become a video game of likelihood, frequently bringing about wasted time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask concerns like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it gets to be to isolate the precise situations less than which the bug happens.
As you’ve collected more than enough data, try to recreate the situation in your local setting. This could indicate inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge scenarios or state transitions concerned. These assessments not only aid expose the situation but also avert regressions Down the road.
Occasionally, The problem can be environment-certain — it'd materialize only on particular working devices, browsers, or under specific configurations. Employing instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It needs endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are presently halfway to fixing it. Using a reproducible situation, You may use your debugging applications extra effectively, test possible fixes safely, and communicate more clearly with your team or buyers. It turns an summary criticism right into a concrete problem — and that’s exactly where developers prosper.
Examine and Fully grasp the Error Messages
Error messages tend to be the most respected clues a developer has when something goes wrong. Rather then observing them as annoying interruptions, developers ought to learn to take care of error messages as direct communications from the process. They generally let you know precisely what transpired, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Begin by reading the concept very carefully As well as in entire. Numerous builders, particularly when under time force, glance at the main line and quickly begin earning assumptions. But deeper in the mistake stack or logs might lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — examine and realize them to start with.
Split the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it issue to a particular file and line selection? What module or perform triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also handy to know the terminology with the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some problems are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context through which the mistake happened. Verify connected log entries, enter values, and up to date changes within the codebase.
Don’t forget about compiler or linter warnings both. These normally precede bigger concerns and supply hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, encouraging you pinpoint issues quicker, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more impressive tools in a developer’s debugging toolkit. When utilized successfully, it provides genuine-time insights into how an application behaves, helping you understand what’s happening underneath the hood without having to pause execution or move in the code line by line.
A very good logging tactic commences with figuring out what to log and at what stage. Frequent logging amounts contain DEBUG, Information, WARN, Mistake, and Deadly. Use DEBUG for in depth diagnostic details throughout improvement, INFO for typical gatherings (like profitable commence-ups), WARN for opportunity difficulties that don’t split the appliance, ERROR for precise challenges, and Deadly when the procedure can’t keep on.
Keep away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure crucial messages and slow down your process. Give attention to key situations, condition changes, enter/output values, and critical conclusion factors in your code.
Structure your log messages clearly and continually. Contain context, which include timestamps, request IDs, and performance names, so it’s simpler to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are satisfied, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about equilibrium and clarity. Using a very well-thought-out logging strategy, you could reduce the time it requires to identify challenges, acquire deeper visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a specialized undertaking—it is a form of investigation. To successfully discover and deal with bugs, builders must method the method just like a detective fixing a thriller. This way of thinking helps break down complicated concerns into manageable areas and observe clues logically to uncover the foundation bring about.
Get started by accumulating proof. Think about the symptoms of the challenge: error messages, incorrect output, or functionality troubles. The same as a detective surveys a criminal offense scene, accumulate just as much appropriate data as it is possible to with no jumping to conclusions. Use logs, examination circumstances, and user reviews to piece together a clear photograph of what’s going on.
Upcoming, sort hypotheses. Question by yourself: What could possibly be leading to this behavior? Have any changes recently been built to your codebase? Has this situation transpired prior to under identical situation? The aim would be to narrow down options and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the challenge within a managed natural environment. Should you suspect a specific purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and Enable the outcome lead you nearer to the truth.
Fork out close notice to tiny details. Bugs generally hide from the least envisioned locations—similar to a missing semicolon, an off-by-just one error, or perhaps a race affliction. Be thorough and client, resisting the urge to patch the issue with no fully comprehension it. Temporary fixes may possibly disguise the real challenge, only for it to resurface later on.
And lastly, maintain notes on That which you tried and realized. Equally as detectives log their investigations, documenting your debugging procedure can preserve time for upcoming concerns and enable others recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical capabilities, solution issues methodically, and grow to be more practical at uncovering concealed issues in sophisticated systems.
Compose Assessments
Crafting tests is one of the best solutions to help your debugging abilities and All round growth performance. Assessments don't just help catch bugs early but additionally serve as a safety net that gives you self-assurance when generating improvements on your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint accurately where and when a problem takes place.
Get started with device assessments, which center on particular person features or modules. These smaller, isolated assessments can speedily reveal regardless of whether a particular piece of logic is working as envisioned. When a test fails, you straight away know where by to glance, appreciably minimizing time invested debugging. Device checks are Specially valuable for catching regression bugs—concerns that reappear following previously remaining fastened.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These enable be certain that different parts of your software perform together efficiently. They’re specifically useful for catching bugs that come about in sophisticated systems with many elements or services interacting. If a thing breaks, your tests can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely think critically regarding your code. To check a attribute properly, you may need to know its inputs, predicted outputs, and edge cases. This standard of understanding Obviously leads to higher code composition and fewer bugs.
When debugging a concern, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the test fails persistently, you could give attention to repairing the bug and check out your check move when The difficulty is resolved. This strategy makes sure that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from a discouraging guessing game into a structured and predictable method—serving to you capture extra bugs, quicker and even more reliably.
Just take Breaks
When debugging a tough difficulty, it’s easy to become immersed in the trouble—watching your display screen for several hours, seeking Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping away. Using breaks will help you reset your brain, lessen annoyance, and infrequently see The problem from the new viewpoint.
When you are also near to the code for also extended, cognitive fatigue sets in. You may begin overlooking apparent errors or misreading code that you wrote just hours before. With this condition, your brain turns into much less effective at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a unique process for 10–quarter-hour can refresh your aim. Quite a few developers report discovering the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done while in the background.
Breaks also help protect against burnout, In particular for the duration of for a longer time debugging classes. Sitting down in front of a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent means that you can return with renewed Vitality along with a clearer mindset. You would possibly out of the blue discover a lacking semicolon, a logic flaw, or maybe a misplaced variable that eluded you ahead of.
In the event you’re trapped, an excellent general guideline is usually to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment split. Use that point to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, nonetheless it actually brings about faster and simpler debugging Ultimately.
In brief, having breaks just isn't an indication of weakness—it’s a wise system. It gives your brain House to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Understand From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to expand being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, more info every one can teach you some thing useful when you take the time to reflect and evaluate what went Mistaken.
Start out by inquiring you a few important queries after the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with better practices like unit tests, code reviews, or logging? The answers often reveal blind places in the workflow or understanding and help you build stronger coding habits moving ahead.
Documenting bugs will also be a wonderful pattern. Continue to keep a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see designs—recurring concerns or typical errors—that you can proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends could be especially impressive. No matter if it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, supporting Other individuals avoid the similar concern boosts team performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are certainly not the ones who produce excellent code, but individuals that constantly study from their errors.
In the long run, Every bug you deal with adds a fresh layer towards your skill established. So next time you squash a bug, take a minute to replicate—you’ll come away a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.